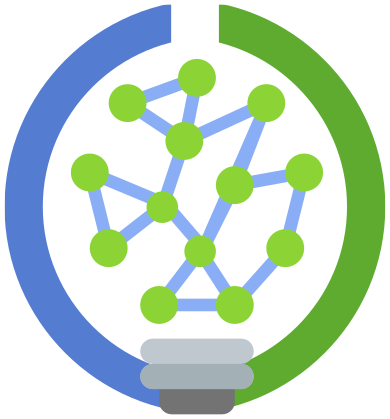
This is part of the Scicloj Clojure Data Scrapbook. |
Clay & Noj demo: image processing
ns index
(:require [tech.v3.libs.buffered-image :as bufimg]
(:as dtype]
[tech.v3.datatype :as tensor]
[tech.v3.tensor :as fun]
[tech.v3.datatype.functional :as kind])) [scicloj.kindly.v4.kind
:style "img {max-width: 100%}"]) (kind/hiccup [
:youtube-id "fd4kjlws6Ts"}) (kind/video {
Arithmetic
+ 1 2) (
3
Loading data
defonce raw-image
(
(bufimg/load"https://upload.wikimedia.org/wikipedia/commons/1/1e/Gay_head_cliffs_MV.JPG"))
type raw-image) (
java.awt.image.BufferedImage
(bufimg/image-type raw-image)
:byte-bgr
Displaying images
raw-image
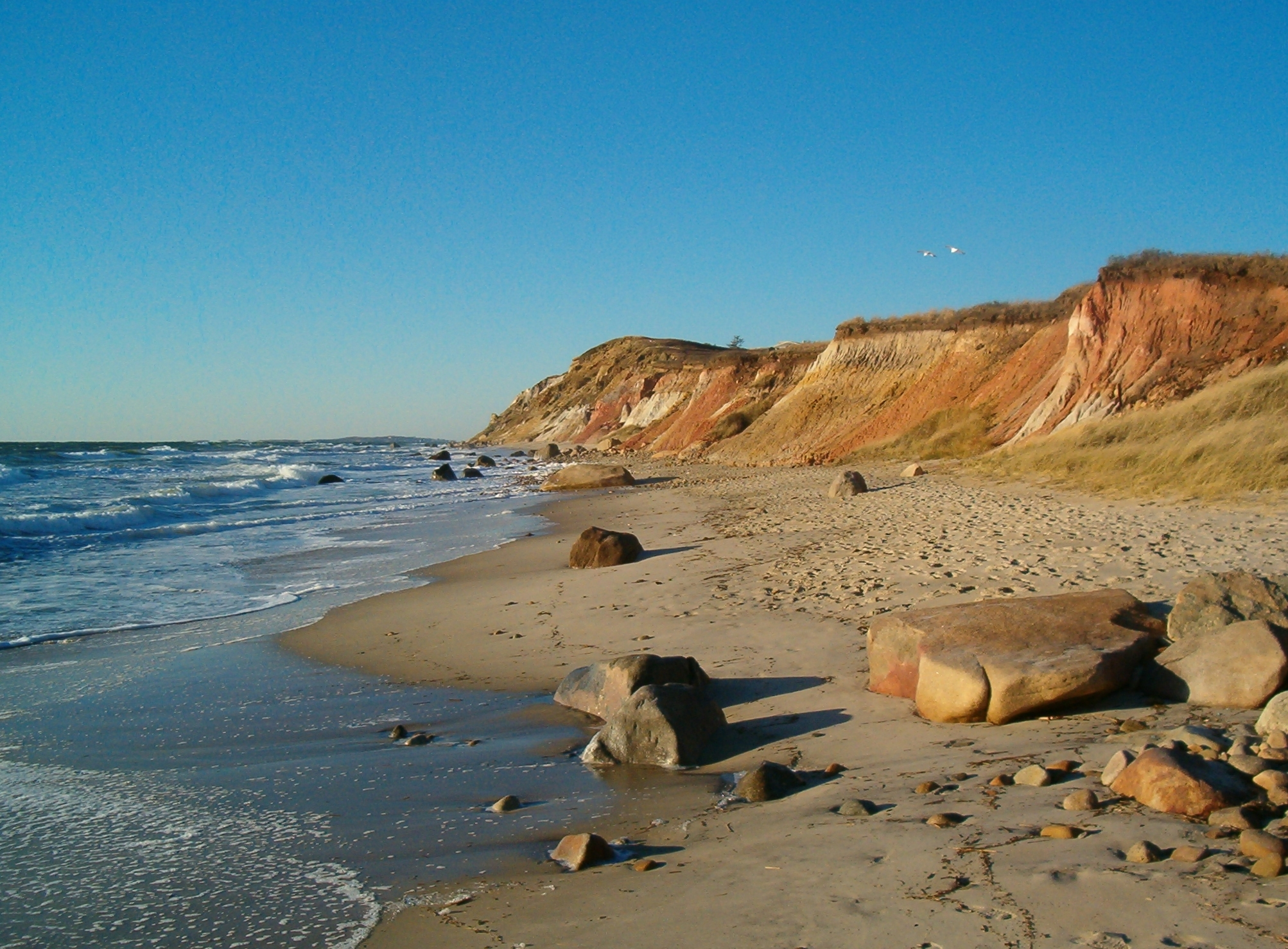
Tensors
defonce raw-tensor
(-> raw-image
( bufimg/as-ubyte-tensor))
(dtype/shape raw-tensor)
1478 2006 3] [
Processing
(kind/fragment
[raw-image-> raw-tensor
(0.5)
(fun/* :byte-bgr))]) (bufimg/tensor->image
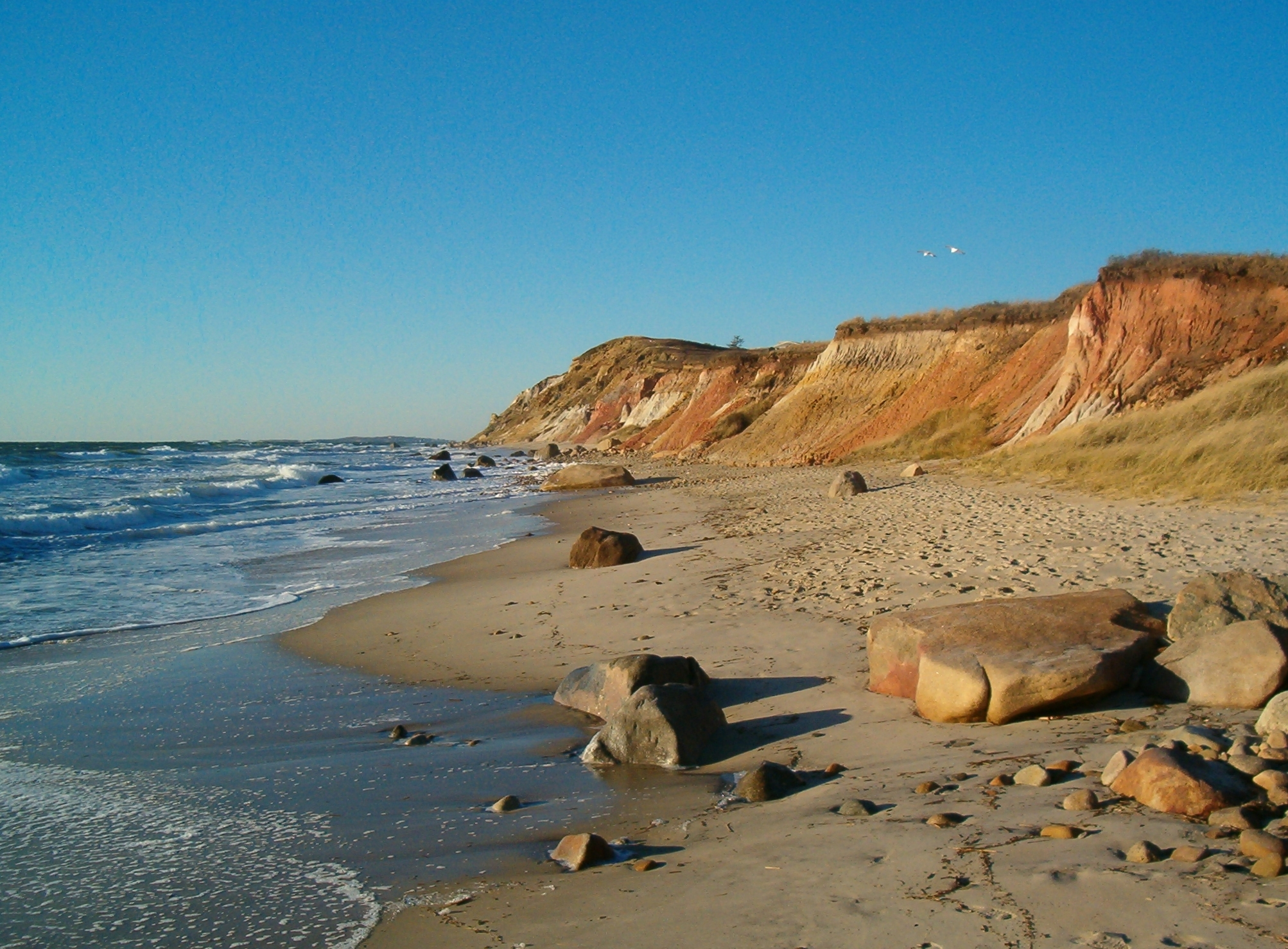
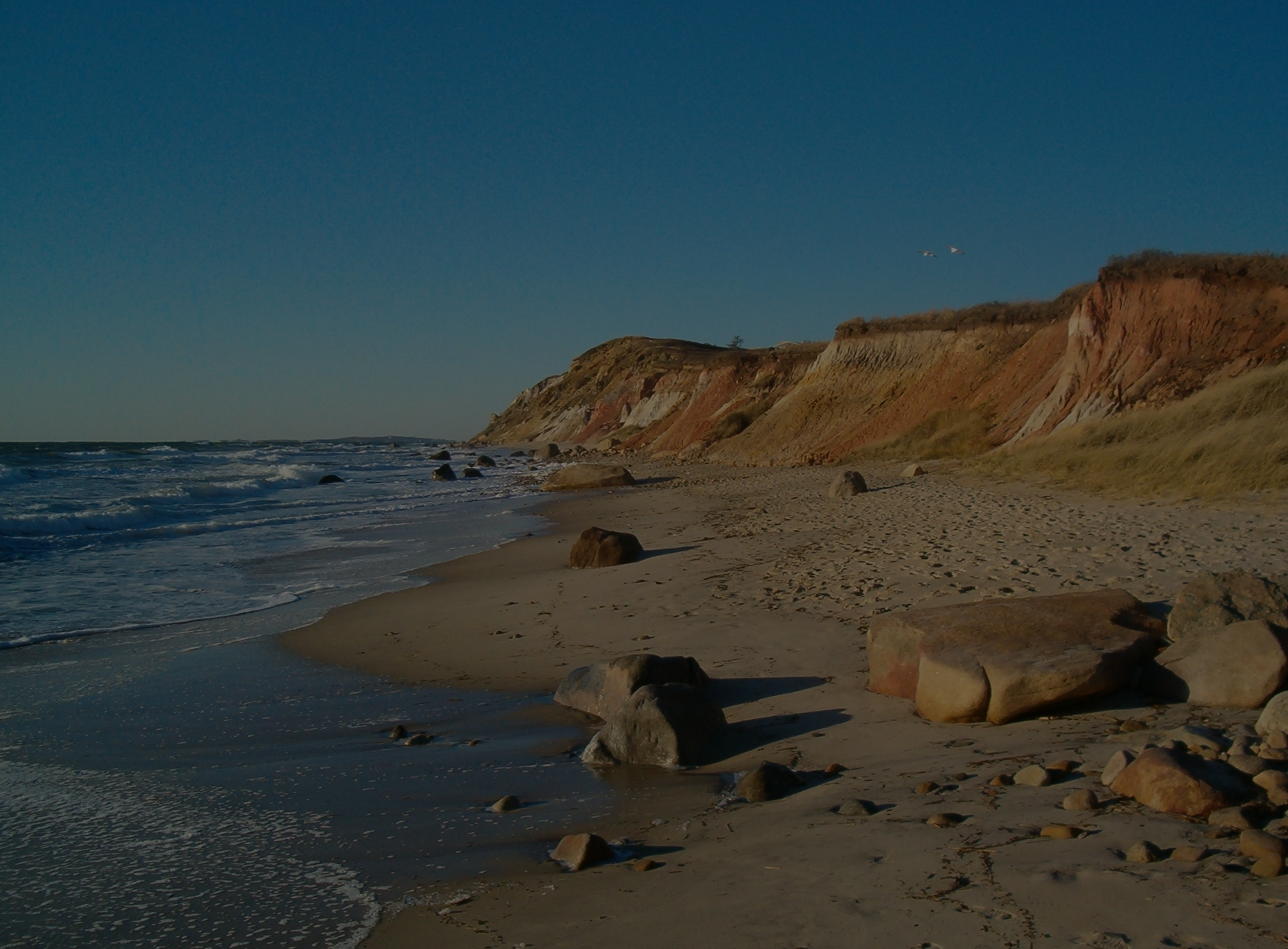
Hiccup
(kind/hiccup:div
[:h3 "raw image"]
[
raw-image:h3 "darkened image"]
[-> raw-tensor
(0.5)
(fun/* :byte-bgr))]) (bufimg/tensor->image
raw image
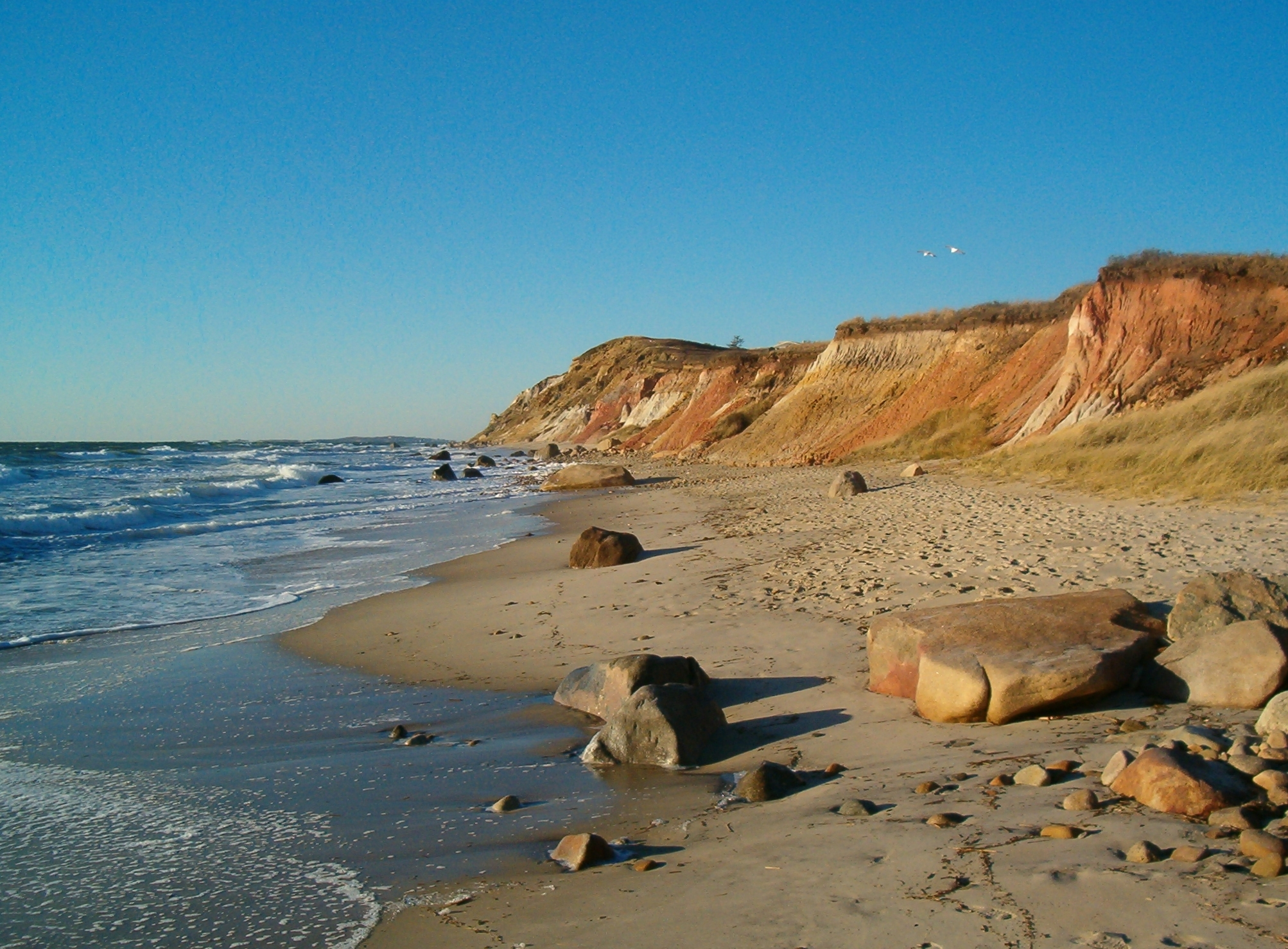
darkened image
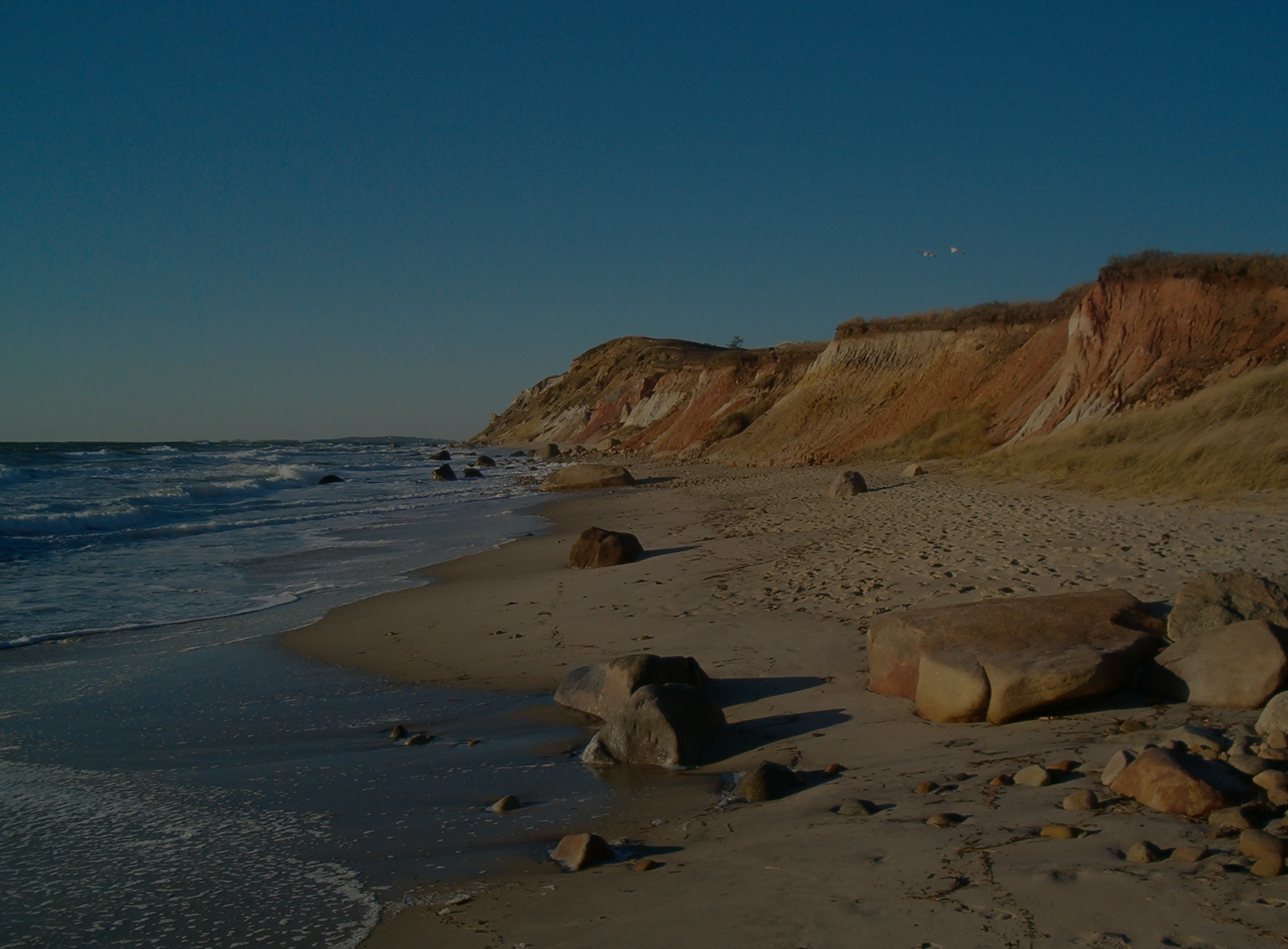
Colour channels
def colour-channels
(-> raw-tensor
(1))) (tensor/slice-right
def blue (colour-channels 0)) (
def green (colour-channels 1)) (
def red (colour-channels 2)) (
count colour-channels) (
3
mapv dtype/shape colour-channels) (
1478 2006] [1478 2006] [1478 2006]] [[
-> (tensor/compute-tensor (dtype/shape raw-tensor)
(fn [i j k]
(if (= k 2)
(
(raw-tensor i j k)0))
:uint8)
:byte-bgr)) (bufimg/tensor->image
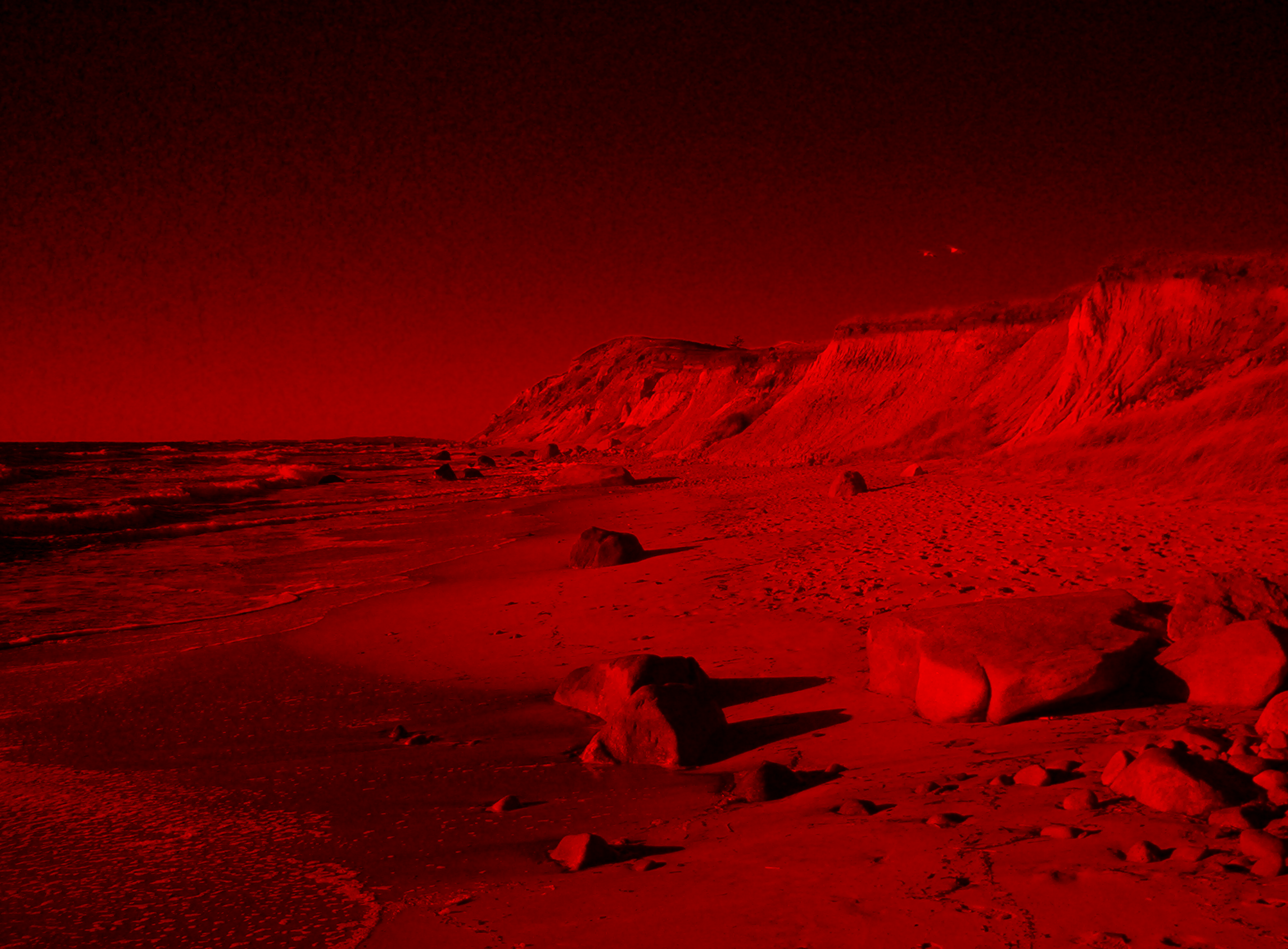
Conditioned processing
-> (tensor/compute-tensor (dtype/shape raw-tensor)
(fn [i j k]
(*
(
(raw-tensor i j k)if (> (green i j)
(
(blue i j))0.3
1)))
:uint8)
:byte-bgr)) (bufimg/tensor->image
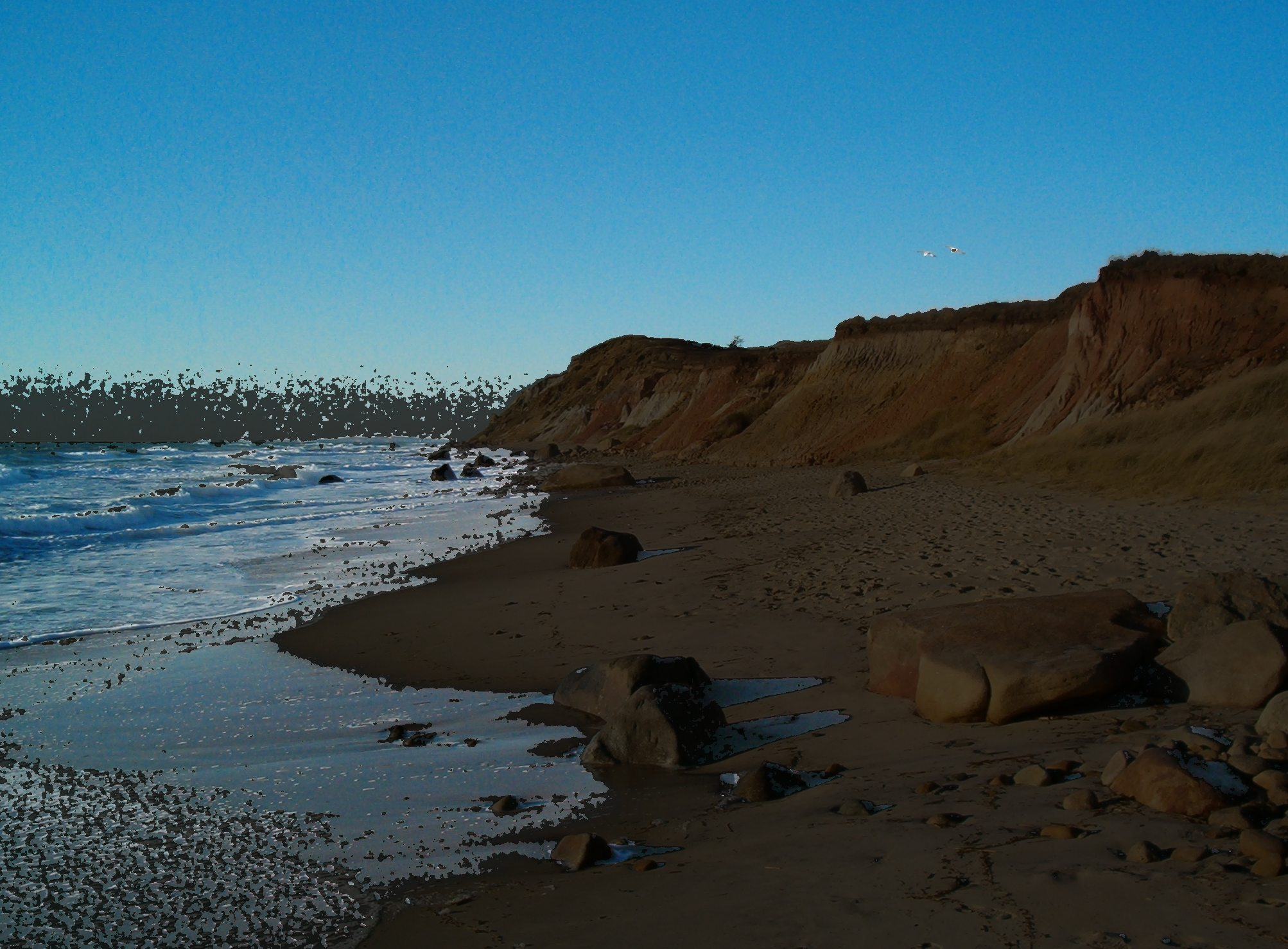
-> (tensor/compute-tensor (dtype/shape raw-tensor)
(fn [i j k]
(*
(
(raw-tensor i j k)if (> (green i j)
(* 1.2 (blue i j)))
(0.3
1)))
:uint8)
:byte-bgr)) (bufimg/tensor->image
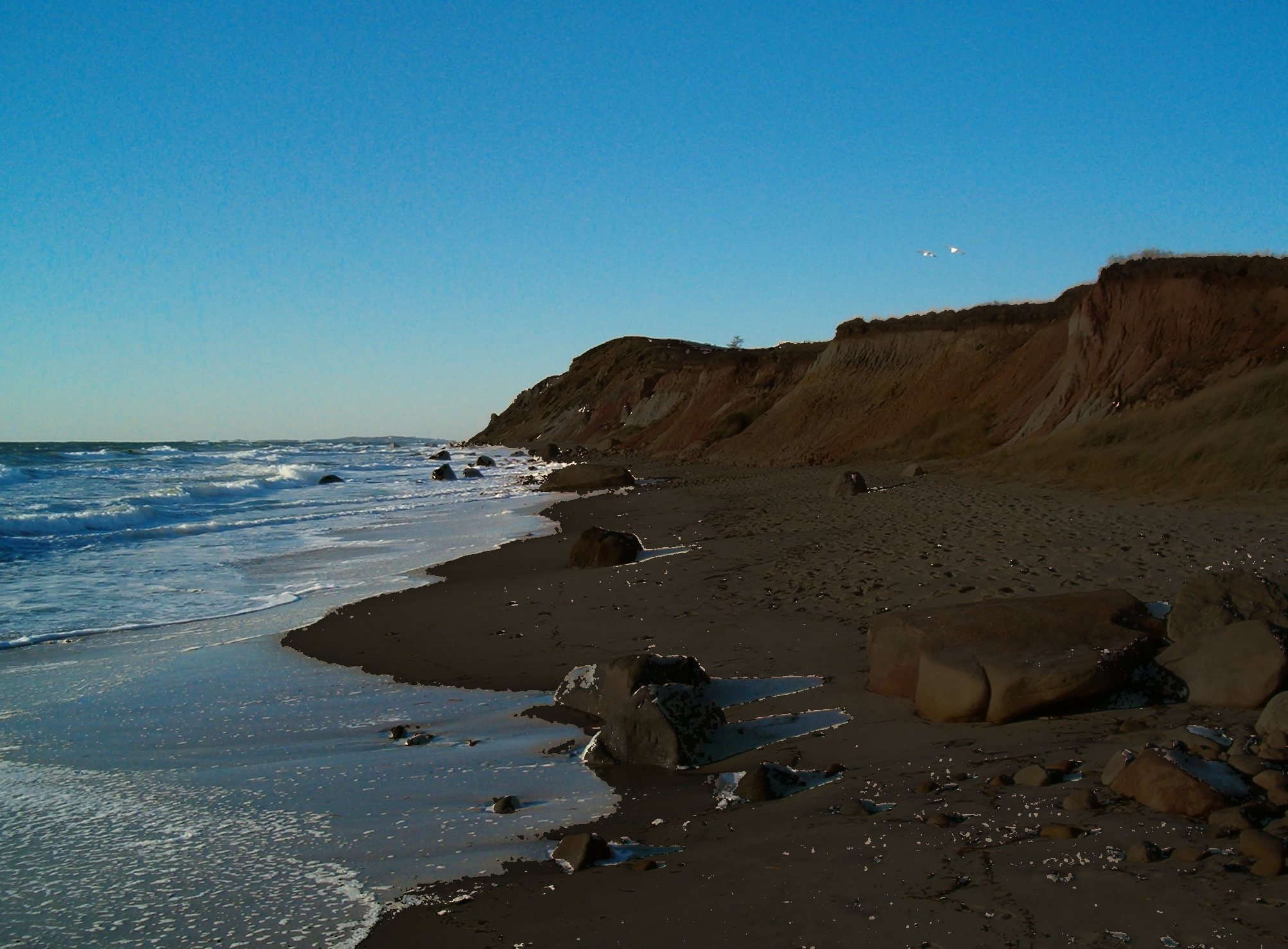
->> [0.7 0.8 0.9 1 1.1 1.2 1.3]
(map (fn [factor]
(
[factor-> (tensor/compute-tensor (dtype/shape raw-tensor)
(fn [i j k]
(*
(
(raw-tensor i j k)if (> (green i j)
(* factor (blue i j)))
(0.3
1)))
:uint8)
:byte-bgr))]))) (bufimg/tensor->image
(
[
0.7
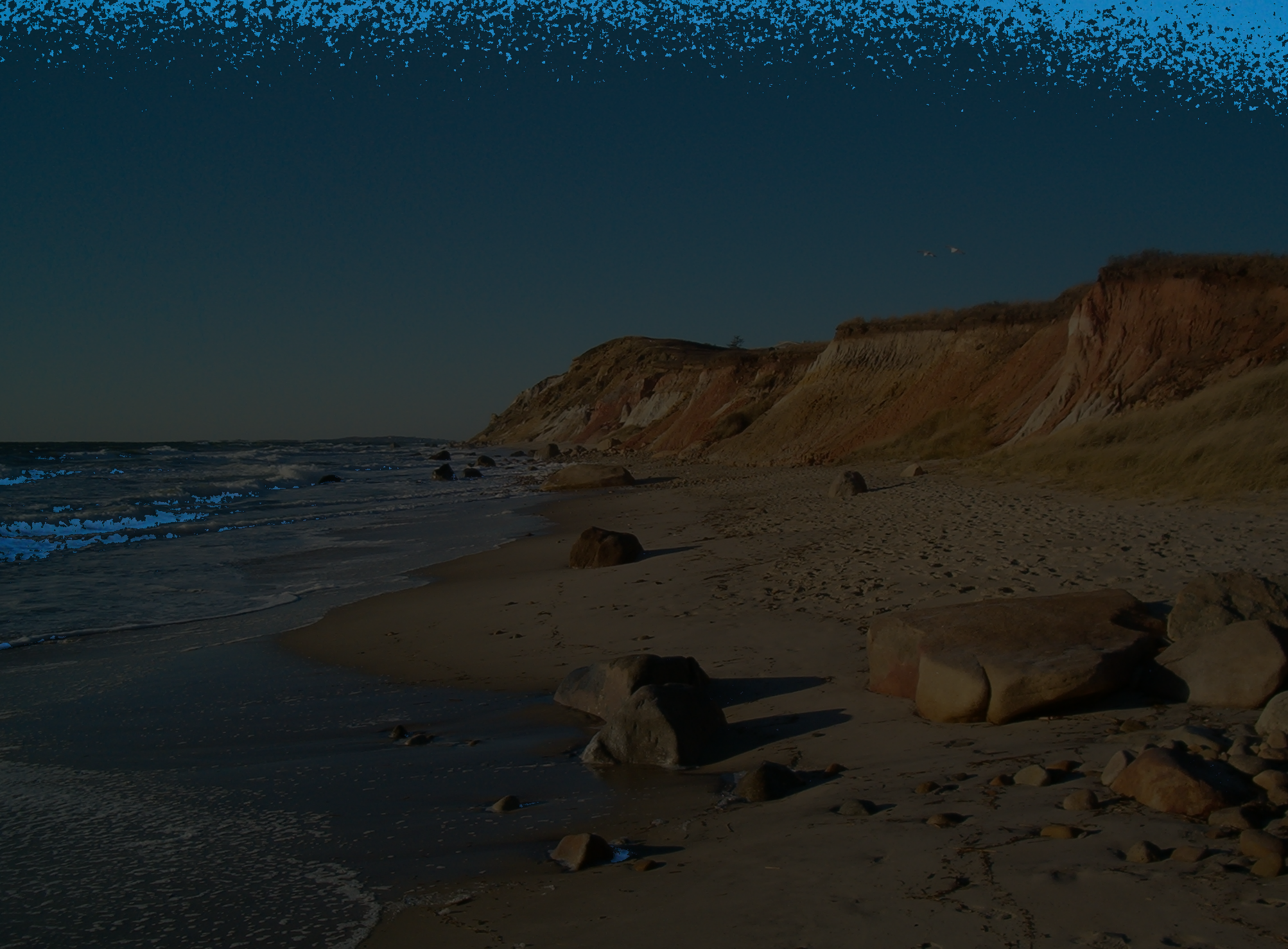
]
[
0.8
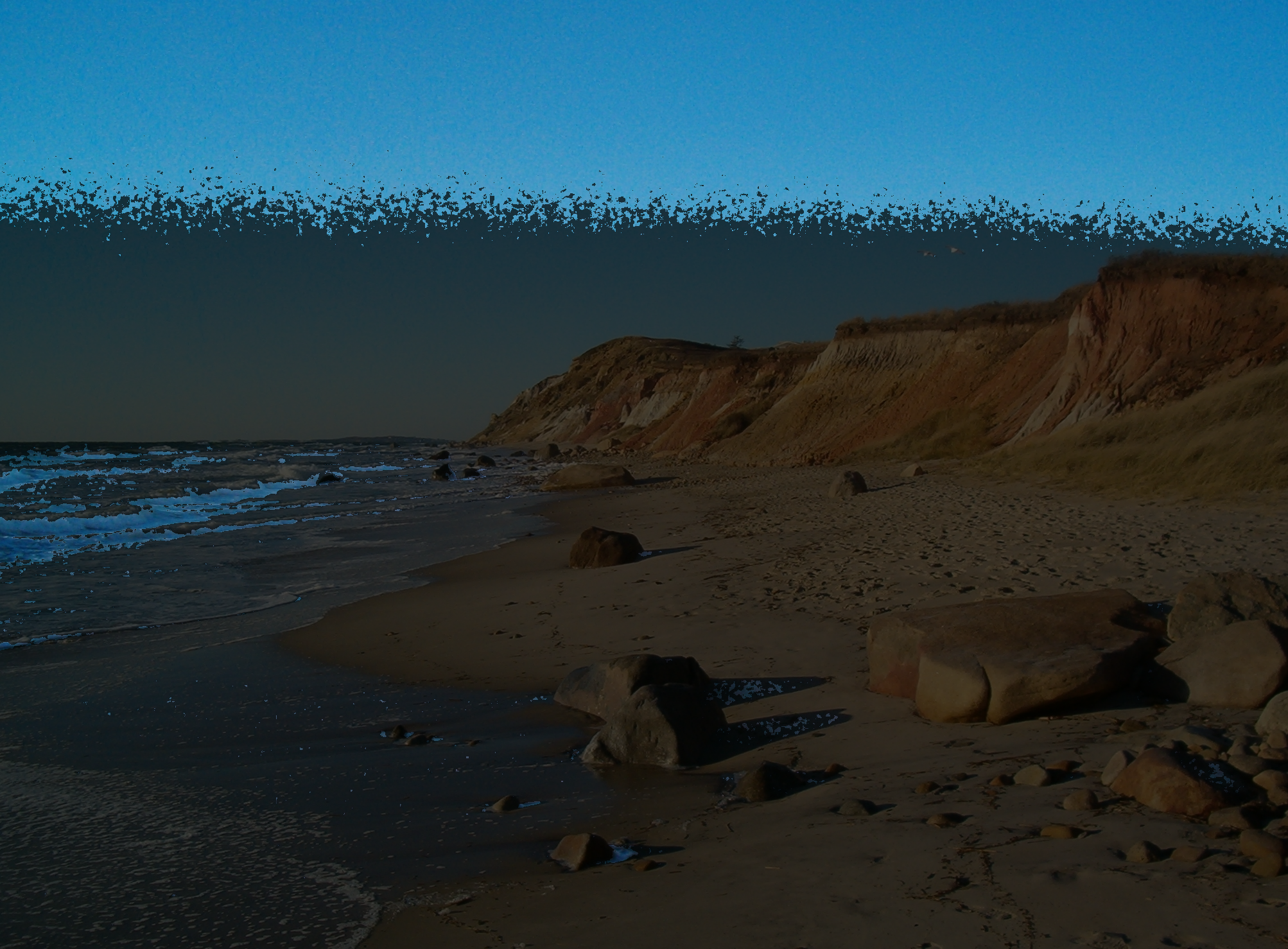
]
[
0.9
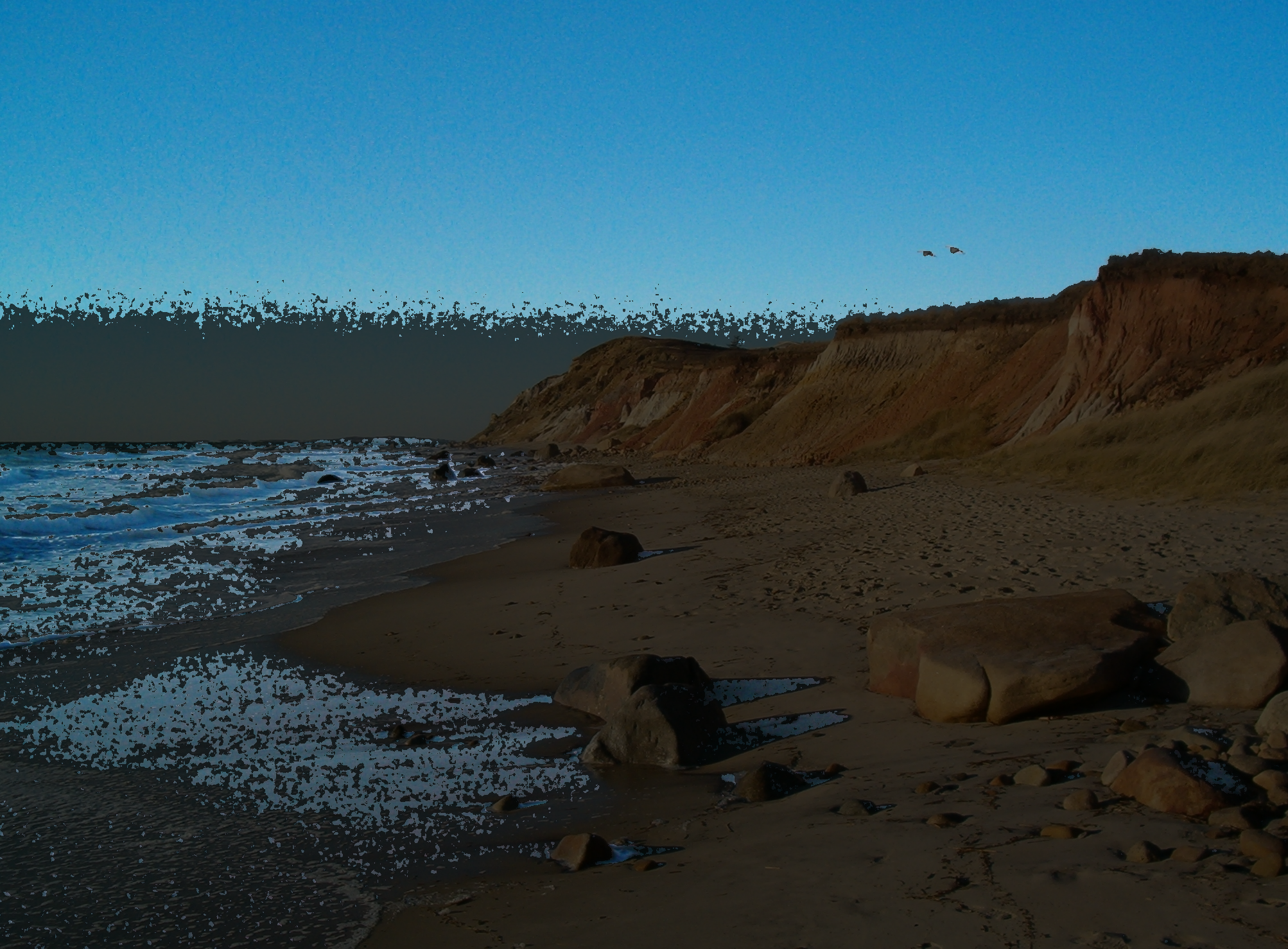
]
[
1
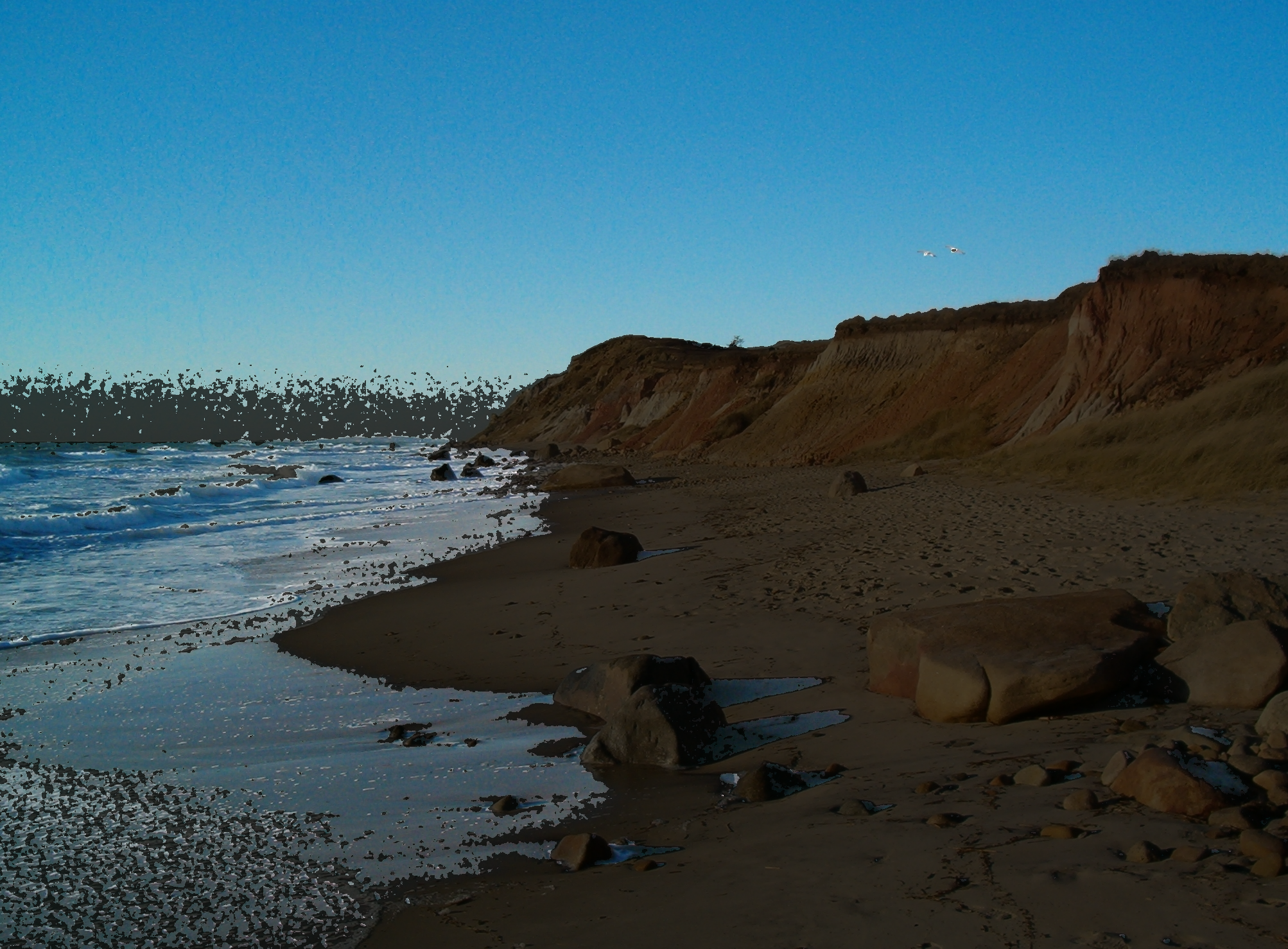
]
[
1.1
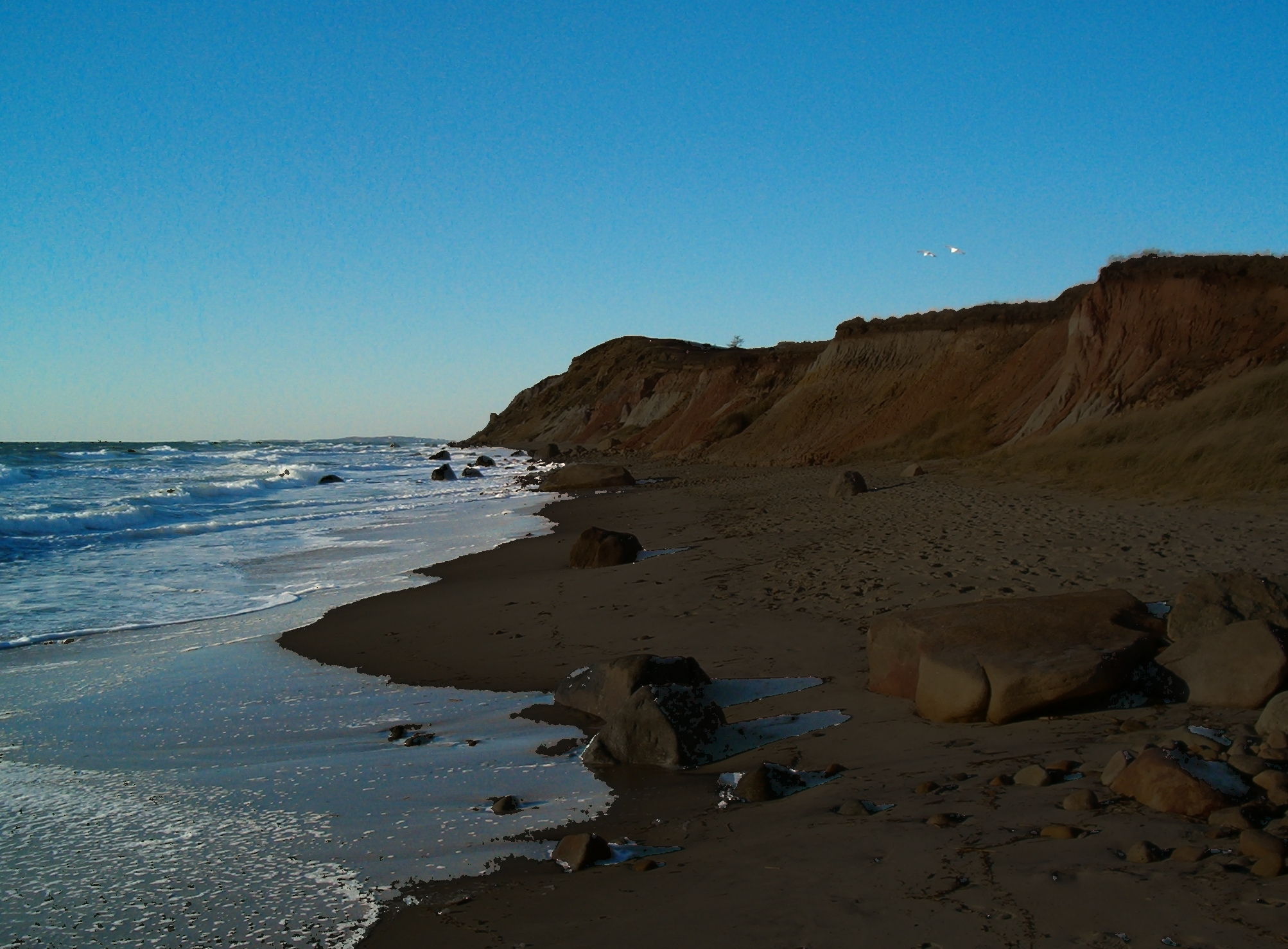
]
[
1.2
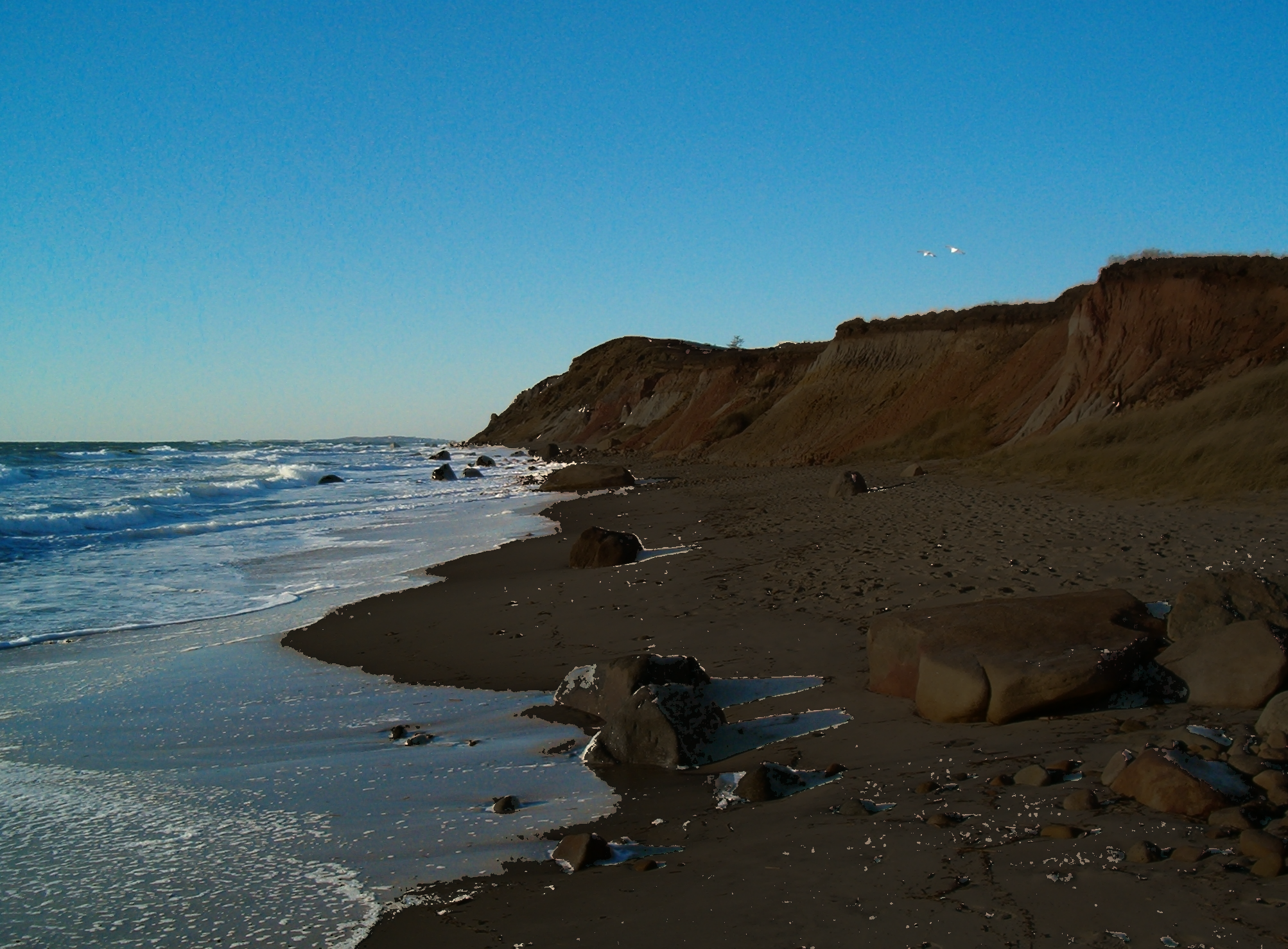
]
[
1.3
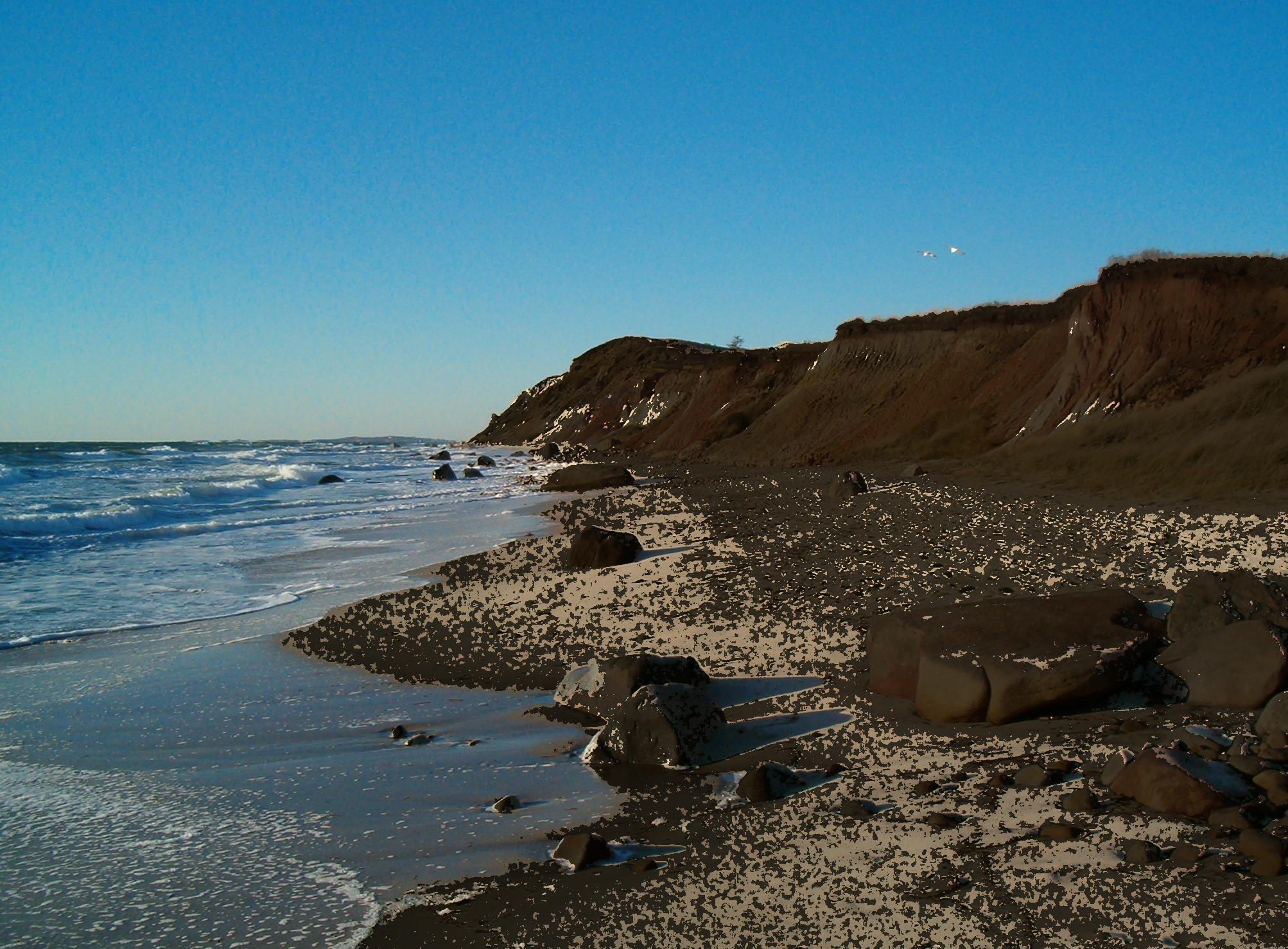
]
)