Wolfram Langauge interop with Wolframite
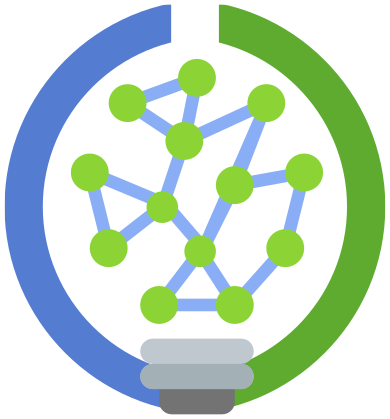
This is part of the Scicloj Clojure Data Scrapbook. |
This notebook demonstrates basic usage of Wolframite in a way that would work in visual tools supporting Kindly. It is also appears at the Wolrfamite repo as dev/kindly-demo.clj. Note that to use Wolframite, you need Wolfamite in the dependencies, and if Wolfraimte fails to find the correct install path automatically, you may need to have the WOLFRAM_INSTALL_PATH
environment variable set up in your system, as explained in Wolframite’s README.
ns index
(:refer-clojure
(;; Exclude symbols also used by Wolfram:
:exclude [Byte Character Integer Number Short String Thread])
:require
(:as wl]
[wolframite.core :refer [view]]
[wolframite.tools.hiccup :as parse]
[wolframite.base.parse
[wolframite.jlink]:as kind]
[scicloj.kindly.v4.kind :as kindly])
[scicloj.kindly.v4.api :import (com.wolfram.jlink MathCanvas KernelLink)
(
(java.awt Color Frame) (java.awt.event WindowAdapter ActionEvent)))
Init (base example)
1 2 3] [4 5 6])) (wl/eval '(Dot [
32
Strings of WL code
"{1 , 2, 3} . {4, 5, 6}") (wl/eval
32
Def / intern WL fns, i.e. effectively define WL fns as clojure fns:
def W:Plus (parse/parse-fn 'Plus {:kernel/link @wl/kernel-link-atom})) (
:Plus 1 2 3) (W
6
… and call it
def greetings
(
(wl/eval"Hello, " x "! This is a Mathematica function's output.")))) '(Function [x] (StringJoin
"Stephen") (greetings
"Hello, Stephen! This is a Mathematica function's output."
Bidirectional translation
(Somewhat experimental, especially in the wl->clj direction)
"GridGraph[{5, 5}]") (wl/->clj!
5 5]) (GridGraph [
5 5]) {:output-fn str}) (wl/->wl! '(GridGraph [
"GridGraph[{5, 5}]"
Graphics
(view5 5])) '(GridGraph [
GridGraph[{5, 5}]
(view5 5])
'(GridGraph [:folded? true}) {
GridGraph[{5, 5}]
(view"Ethanol" "StructureDiagram")) '(ChemicalData
ChemicalData["Ethanol", "StructureDiagram"]
More Working Examples
"Ocean") Here)) (wl/eval '(GeoNearest (Entity
:entity.ocean/mediterranean-sea-eastern-basin
[:entity.ocean/mediterranean-sea]
TODO: Make this work with view
as well.
"The cat sat on the mat.")) (view '(TextStructure
TextStructure["The cat sat on the mat."]
Wolfram Alpha
"number of moons of Saturn" "Result")) (wl/eval '(WolframAlpha
145 (IndependentUnit "moons")) (Quantity
"number of moons of Saturn" "Result")) (view '(WolframAlpha